Smart Habitat Module
Concept
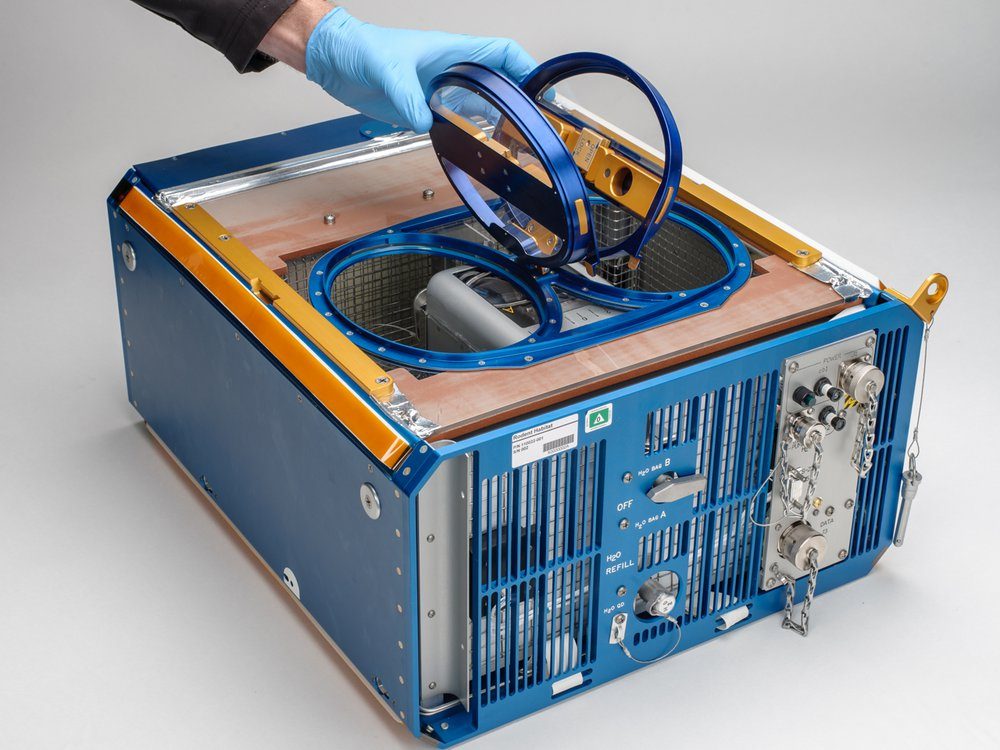
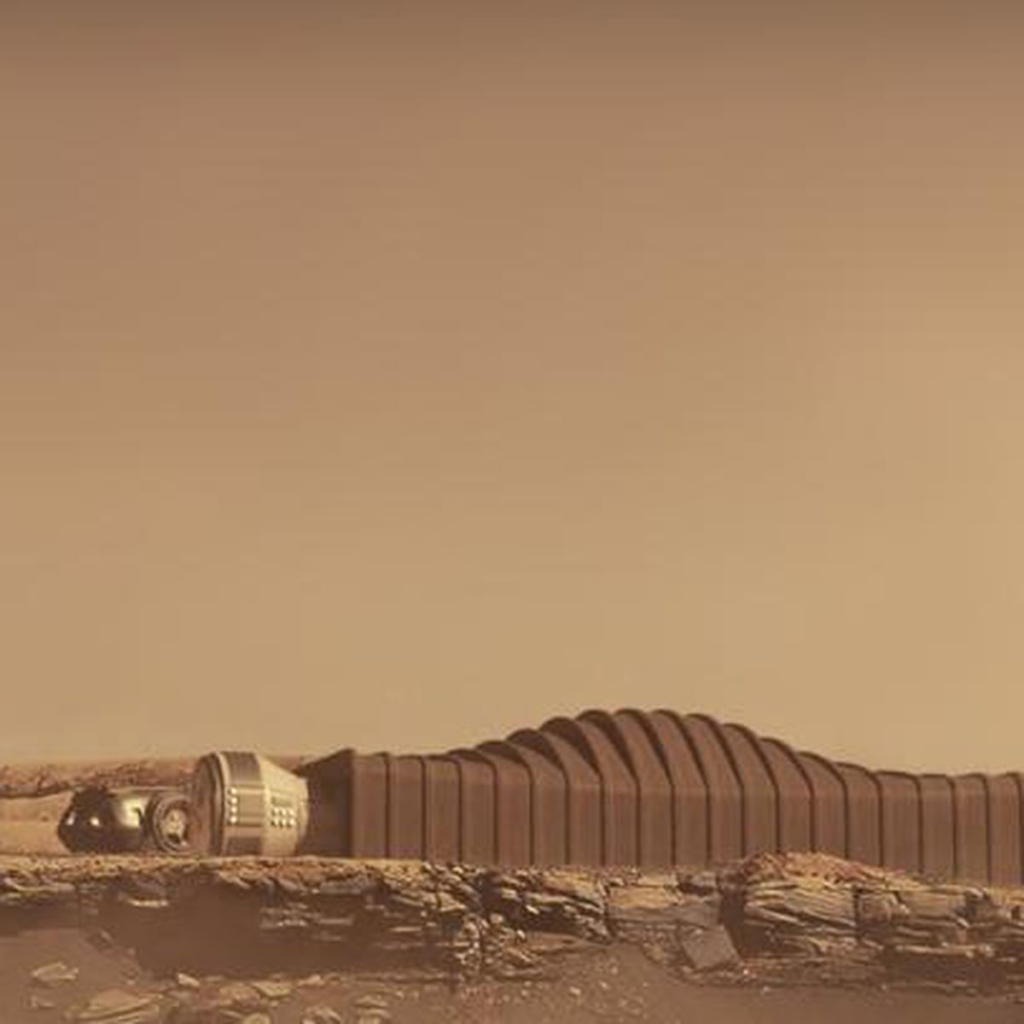
Introduction
As we all know, the environment on Mars is extreme and definitely not suitable for humans or any living things. Therefore, it is important to have a Smart Habitat Module that is installed in the shelter for any human beings to survive through the ever-changing surface of Mars which has a huge difference between day and night. To have a sustainable living environment, comfortableness is a must. Therefore, the astronaut gets to adjust the threshold of the module sensors.
Moreover, since it is a film prop, it would be more realistic if the actor has to interact with it in order to get it to start working. In that case, I will leave the potentiometers detached at first so that the actor has to assemble the potentiometers and find the place on the module to plug them in to start adjusting the threshold. Add some customizability to a such high-tech module with modes On, Auto, and Off all based on whether the potentiometer is rotated to the left, the middle, or the right.
So, what exactly is it and how can we make it?
The Smart Habitat Module is a large, modular box made of ‘durable materials’, designed to serve as both a shelter and a resource-gathering and management center for the stranded astronaut. The module would have an array of built-in sensors, including light [photoresistor] & temperature sensors [DHT11] connected to a hidden Arduino system.
In terms of aesthetics, the module could be designed to look like a futuristic piece of equipment, with clean lines and an attractive design. The Arduino system would be hidden within the walls of the module, making it as unobtrusive as possible while still being fully functional.
The Arduino would be programmed to use these inputs to adjust the module’s systems to optimize conditions for survival. For example, if the light sensor detects a drop in ambient light levels, the module could automatically adjust its lighting system to compensate. If the temperature sensors detect a drop/rise in temperature, the module could activate its fan system to keep the living environment suitable for the astronaut.
The module would also have a number of outputs, such as motors, fans, and LED lights, that could be used to control and manage the various systems within the module. For example, fans could be used to circulate air and regulate temperature, and lights can be used to guarantee the light source. Moreover, the astronaut will be able to adjust the temperature threshold according to his somatosensory.
In conclusion, by using this re-imagined technology, the stranded astronaut would have a more sustainable and efficient means of surviving on Mars, as the module would be able to constantly adjust to the changing conditions on the planet and provide the necessary support for survival.
Tutorials
Requirements
- Arduino Uno
- DHT11 Temperature and Humidity Module
- Fan Balde and 3-6V Motor
- L293D
- Breadboard
- Jumper wires
- LED
- Resistors
- Photoresistor (Photocell)
- Potentiometer 10K * 2
- Power Module
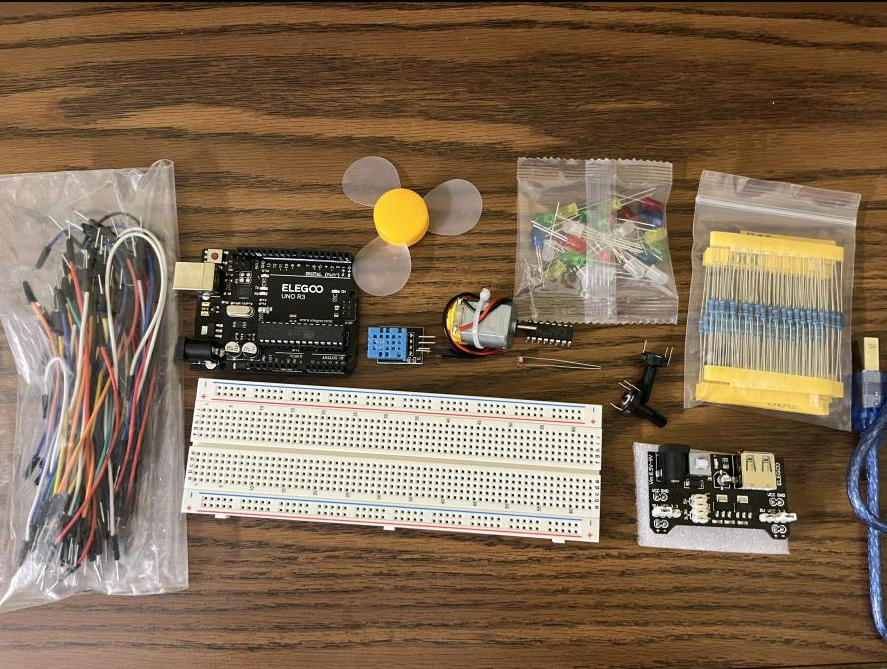
Step 1: Plug in the DHT11 module and test it with the serial reader
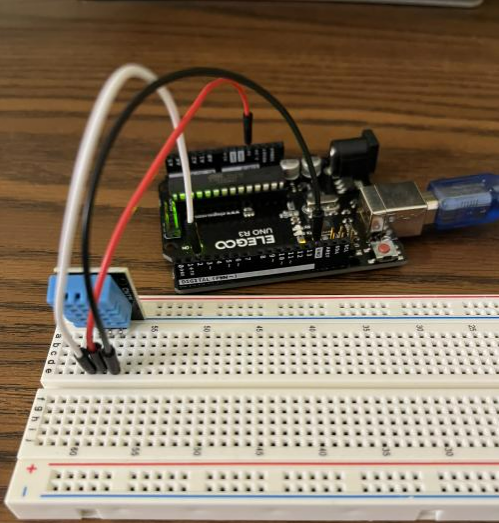
Code
Install the DHT library beforehand
#include “DHT.h”
#define DHTPIN 2 // Analog Pin sensor is connected to
#define DHTTYPE DHT11
// Initialize DHT sensor
DHT dht(DHTPIN, DHTTYPE);
void setup(){
Serial.begin(9600);
// start dht sensor
dht.begin();
}
void loop(){
//Start of Program
Serial.print(“Current humidity = “);
Serial.print(dht.readHumidity());
Serial.print(“% “);
Serial.print(“temperature = “);
Serial.print(dht.readTemperature());
Serial.println(“C “);
delay(2000);//Wait 5 seconds before accessing sensor again.
//Fastest should be once every two seconds.
}// end loop()

Step 2: Plug in the Photoresistor and a resistor, and test it with the serial reader
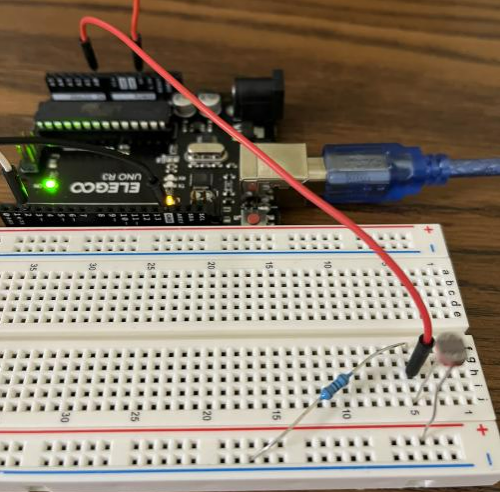
Code
#define PHOTORESISTOR_PIN A0
void setup(){
Serial.begin(9600);
}
loop() {
Serial.println(analogRead(PHOTORESISTOR_PIN));
}
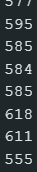
Step 3: Plug in the LED light and a resistor to test the change in the LED light intensity
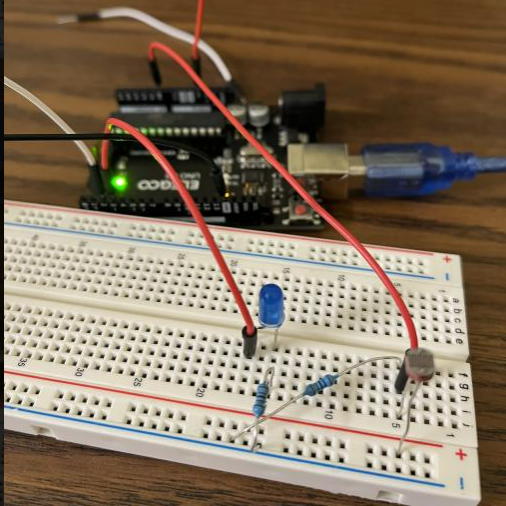
Code
int led = 6; // the PWM pin the LED is attached to
int brightness = 0; // how bright the LED is
int fadeAmount = 5; // how many points to fade the LED by
// the setup routine runs once when you press reset:
void setup() {
// declare pin 9 to be an output:
pinMode(led, OUTPUT);
}
// the loop routine runs over and over again forever:
void loop() {
// set the brightness of pin 9:
analogWrite(led, brightness);
// change the brightness for next time through the loop:
brightness = brightness + fadeAmount;
// reverse the direction of the fading at the ends of the fade:
if (brightness <= 0 || brightness >= 255) {
fadeAmount = -fadeAmount;
}
// wait for 30 milliseconds to see the dimming effect
delay(50);
}
Demo
Step 4: Plug in the L293D, connect the fan blade to the motor and test the functionality
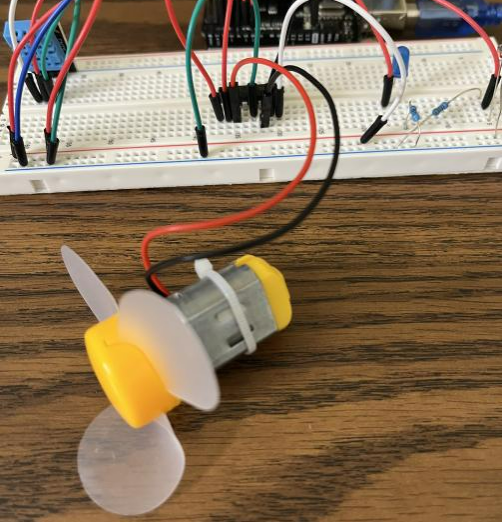
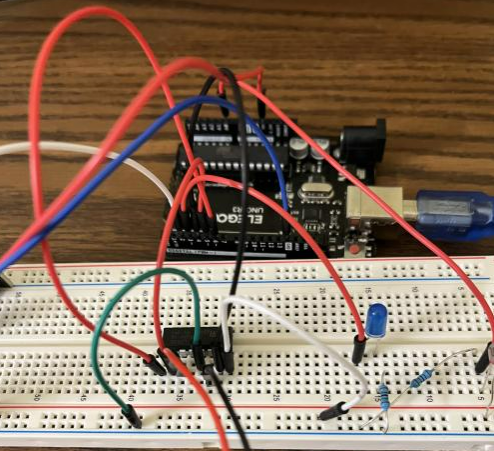
Code
#define DIRA 4
#define DIRB 5
#define ENABLE 6
void setup() {
pinMode(ENABLE,OUTPUT);
pinMode(DIRA,OUTPUT);
pinMode(DIRB,OUTPUT);
}
void loop() {
digitalWrite(DIRA,HIGH); //one way
digitalWrite(DIRB,LOW);
analogWrite(ENABLE,255); //enable on
delay(2000);
}
Demo
Step 5: Adjust the code to connect the reading of the Photoresistor to the light intensity of the LED and test the functionality
Code
Adjust in void loop()
// Photoresistor
int envLight = analogRead(PHOTORESISTOR_PIN);
Serial.println(envLight);
// LED Brightness
analogWrite(LED, brightness);
if (envLight >= 550) {
if (brightness > 0) {
brightness = brightness – fadeAmount;
}
}
else {
if (brightness < 255) {
brightness = brightness + fadeAmount;
}
delay(100);
Demo
Step 6: Adjust the code to connect the reading of the DHT11 module to the rotation speed of the fan blade and test the functionality
Code
int speed = 0;
void loop() {
// DHT11
Serial.print(“temperature = “);
int temperature = dht.readTemperature();
Serial.print(temperature);
Serial.println(“C “);
// Fanblade
if (temperature >= 25) {
speed = 255;
}
else {
speed = 225;
}
digitalWrite(DIRA,LOW); //one way
digitalWrite(DIRB,HIGH);
analogWrite(ENABLE,speed); //enable on
}
delay(100);
Demo
Step 7: Plug in the potentiometers and add the related code to check if it can change the fan speed & LED light intensity
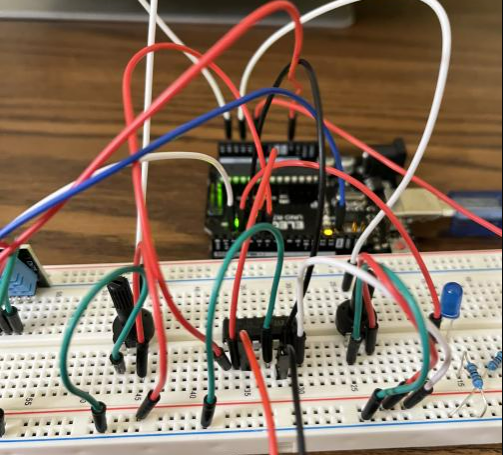
Code Adjusted for Fan Control
#define ANALOGFAN A4
int brightness = 0;
int fadeAmount = 5;
int speed = 180;
void loop() {
// Fanblade
int fanControl = analogRead(ANALOGFAN);
if (fanControl >= 700) {
speed = fanControl/4;
}
else if (temperature >= 25) {
speed = 255;
}
else {
speed = 180;
}
digitalWrite(DIRA,LOW); //one way
digitalWrite(DIRB,HIGH);
analogWrite(ENABLE,speed); //enable on
}
Demo
Code Adjusted for LED Control
#define ANALOGLED A2
void loop() {
// Photoresistor
int envLight = analogRead(PHOTORESISTOR_PIN);
int ledControl = analogRead(ANALOGLED);
// LED Brightness
if (ledControl >= 700) {
if (brightness < 255) {
brightness = brightness + fadeAmount;
}
}
else if (envLight >= 550) {
if (brightness > 0) {
brightness = brightness – fadeAmount;
}
}
else {
if (brightness < 255) {
brightness = brightness + fadeAmount;
}
}
analogWrite(LED, brightness);
}
Demo
Step 8: Combine everything together and test the functionality
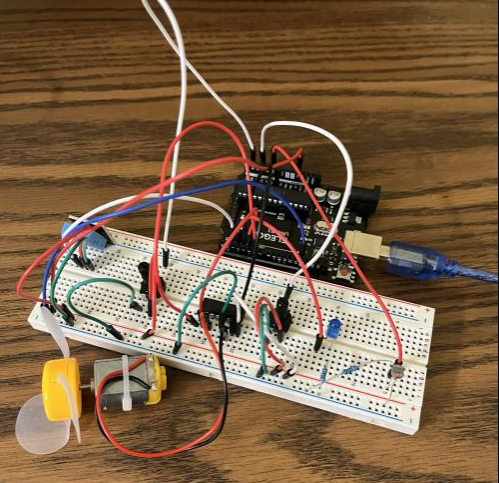
Demo
Step 8.5: The power from Arduino Uno itself is not enough for the fan blade to reach its ideal speed, add a power module to it and rewire
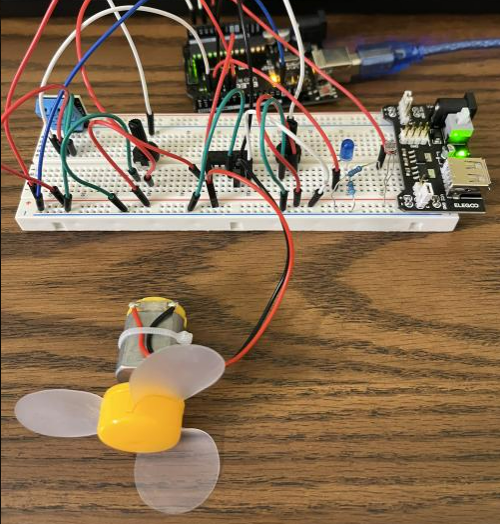
Demo
Step 9: Encapsulation
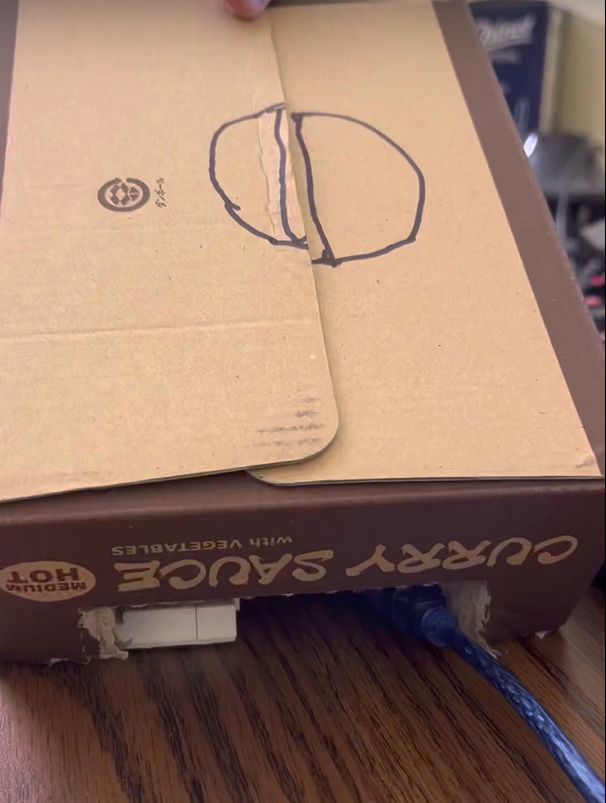

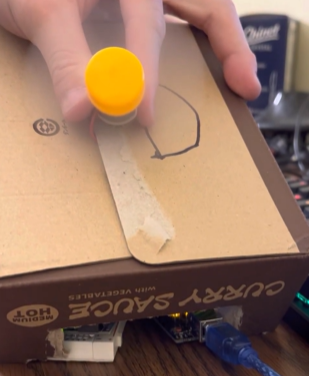
Code
#include “DHT.h”
#define DHTPIN 2 // Analog Pin sensor is connected to
#define DHTTYPE DHT11
#define PHOTORESISTOR_PIN A0
#define LED 3
#define DIRA 4
#define DIRB 5
#define ENABLE 6
#define ANALOGFAN A4
#define ANALOGLED A2
int brightness = 0;
int fadeAmount = 5;
int speed = 180;
// Initialize DHT sensor
DHT dht(DHTPIN, DHTTYPE);
void setup(){
Serial.begin(9600);
// LED
pinMode(LED, OUTPUT);
// Start DHT Sensor
dht.begin();
// Fanblade
pinMode(ENABLE,OUTPUT);
pinMode(DIRA,OUTPUT);
pinMode(DIRB,OUTPUT);
}
void loop(){
//Start of Program
// DHT11
Serial.print(“temperature = “);
int temperature = dht.readTemperature();
Serial.print(temperature);
Serial.println(“C “);
// Fanblade
int fanControl = analogRead(ANALOGFAN);
if (fanControl >= 700) {
speed = 255;
}
else if (fanControl <= 100) {
speed = 0;
}
else if (temperature >= 25) {
speed = 255;
}
else {
speed = 160;
}
digitalWrite(DIRA,LOW); //one way
digitalWrite(DIRB,HIGH);
analogWrite(ENABLE,speed); //enable on
// Photoresistor
int envLight = analogRead(PHOTORESISTOR_PIN);
int ledControl = analogRead(ANALOGLED);
// LED Brightness
if (ledControl >= 800) {
if (brightness < 255) {
brightness = brightness + fadeAmount;
}
}
if (ledControl <= 100) {
if (brightness > 0) {
brightness = brightness – fadeAmount;
}
}
else if (envLight >= 550) {
if (brightness > 0) {
brightness = brightness – fadeAmount;
}
}
else {
if (brightness < 255) {
brightness = brightness + fadeAmount;
}
}
analogWrite(LED, brightness);
delay(100);
}// end loop()
Demo
Conclusion
With the help of external tutorials, I am available to understand the functions of each module/component. Since those tutorials only demonstrate how each of them works individually, it is important to also have a good understanding of the principles behind them so that you can express your creativity through manipulating the code to make them function collaboratively at the same time to achieve high functionality as a real Smart Habitat Module.
What worked? What didn’t work?
The threshold adjustment worked perfectly; however, the sensors are not receiving the environmental input accurately. Nevertheless, it works under the extreme, ever-changing environment on Mars. Moreover, the power was not enough to allow the fan blade to reach the ideal rotation speed, therefore, I add a power module to it.
Leave a Reply