Inputs/Outputs
Inputs
- DHT-11 Temperature and Humidity Sensor
- Keyestudio Tilt Sensor
Outputs
- 16×2 Liquid Crystal Display
- Piezzo Buzzer
Description
My film prop project is a thermostat meant that would regulate the temperature of its environment, but with a twist: the thing won’t run properly for more than a few seconds before you need to tilt it around to repair it.
The thermostat uses the DHT-11 temperature and humidity sensor to detect the current temperature. If the target temperature (set in the code) is greater than the target temperature, the thermostat starts to run.
The operation of the thermostat is represented by noise made using a piezzo buzzer. When the thermostat is running, it plays a continuous high-pitched noise. When it breaks, it instead plays short bursts of noise on a specific interval. To repair it, you must tilt it quickly back and forth when one of the short bursts of sound occurs. The first time you do this, the duration of the sound becomes shorter. The second time you tilt it successfully, the thermostat returns to the working state until it breaks again after a random amount of time.
Images
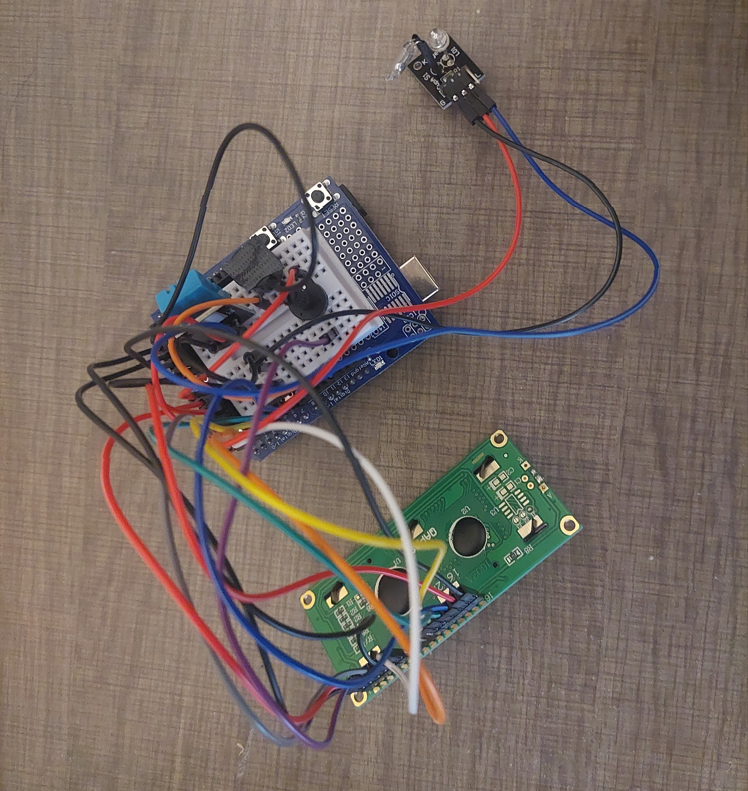
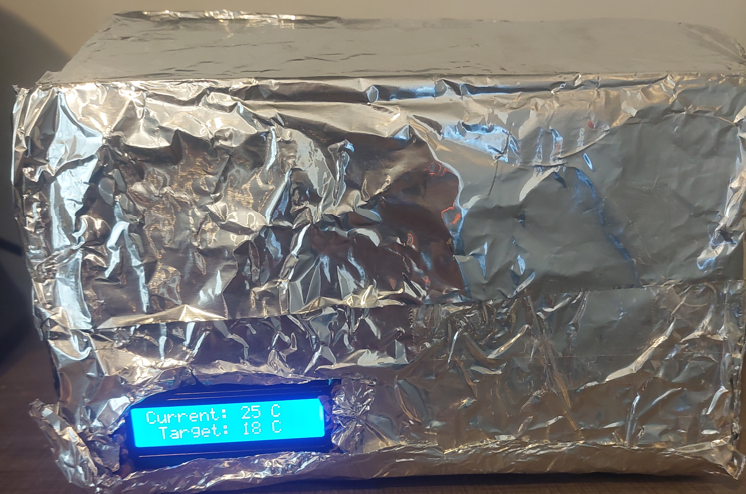
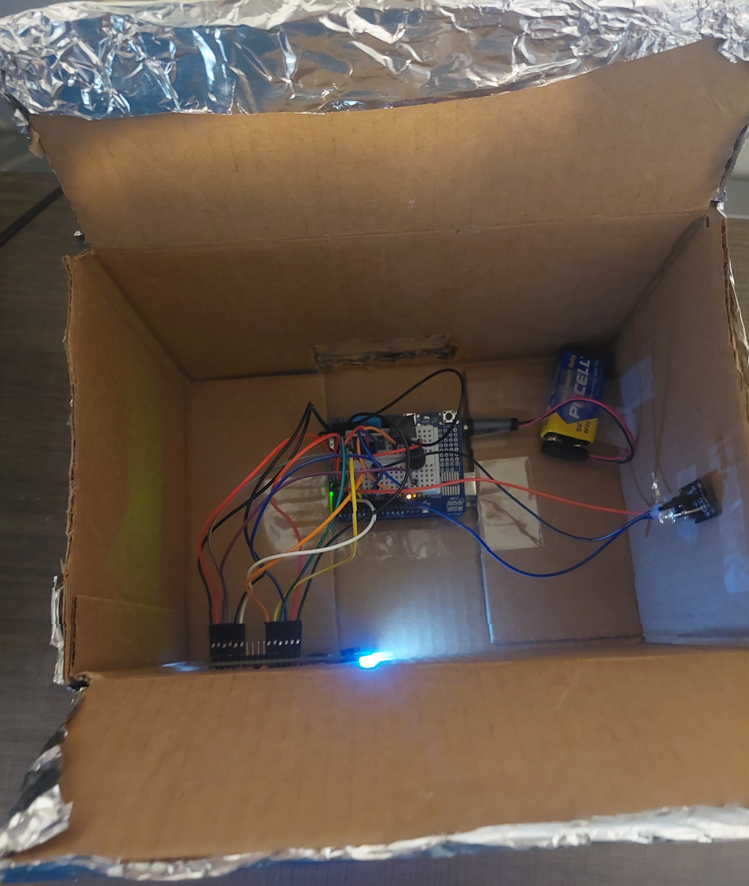
Code and Wiring Diagram
#include <LiquidCrystal.h>
#include <dht.h>
#include "pitches.h"
#define DHT_INPUT 13
#define BUZZER_OUTPUT 12
#define TILT_INPUT 9
LiquidCrystal lcd(1, 2, 4, 5, 6, 7);
dht DHT;
int currentTemperature = 30;
int targetTemperature = 18;
int brokenSoundTimer = 0;
bool isBroken = false;
int iterationsUntilBecomingBroken = 50;
int currentTiltInput = 0;
int previousTiltInput = 0;
int brokenSoundDuration = 375;
void setup() {
lcd.begin(16,2);
pinMode(BUZZER_OUTPUT, OUTPUT);
printTemperature();
}
void loop() {
previousTiltInput = currentTiltInput;
currentTiltInput = digitalRead(TILT_INPUT);
lcd.begin(16,2);
currentTemperature = readCurrentTemperature();
printTemperature();
analogWrite(A2, currentTemperature > targetTemperature ? 255 : 0);
handleBreaking();
if (currentTemperature > targetTemperature) {
if (isBroken) {
if (brokenSoundTimer < 3) {
if (currentTiltInput == HIGH && previousTiltInput != HIGH) {
brokenSoundDuration -= 75;
if (brokenSoundDuration <= 75) {
isBroken = false;
iterationsUntilBecomingBroken = 50 + random() % 100;
}
}
}
if (brokenSoundTimer % 12 == 0) {
tone(BUZZER_OUTPUT, NOTE_D1, brokenSoundDuration);
brokenSoundTimer = 0;
}
brokenSoundTimer++;
} else {
tone(BUZZER_OUTPUT, NOTE_B0, 75);
}
}
delay(100);
}
void handleBreaking() {
if (iterationsUntilBecomingBroken > 0) {
iterationsUntilBecomingBroken--;
if (iterationsUntilBecomingBroken == 0) {
isBroken = true;
brokenSoundDuration = 300;
}
}
}
void printTemperature() {
lcd.setCursor(0, 0);
char buffer[25];
sprintf(buffer, "Current: %d C", currentTemperature);
lcd.print(buffer);
lcd.setCursor(1, 1);
char buffer2[25];
sprintf(buffer, "Target: %d C", targetTemperature);
lcd.print(buffer);
}
int readCurrentTemperature() {
int readTemperature = DHT.read11(DHT_INPUT);
return DHT.temperature;
}
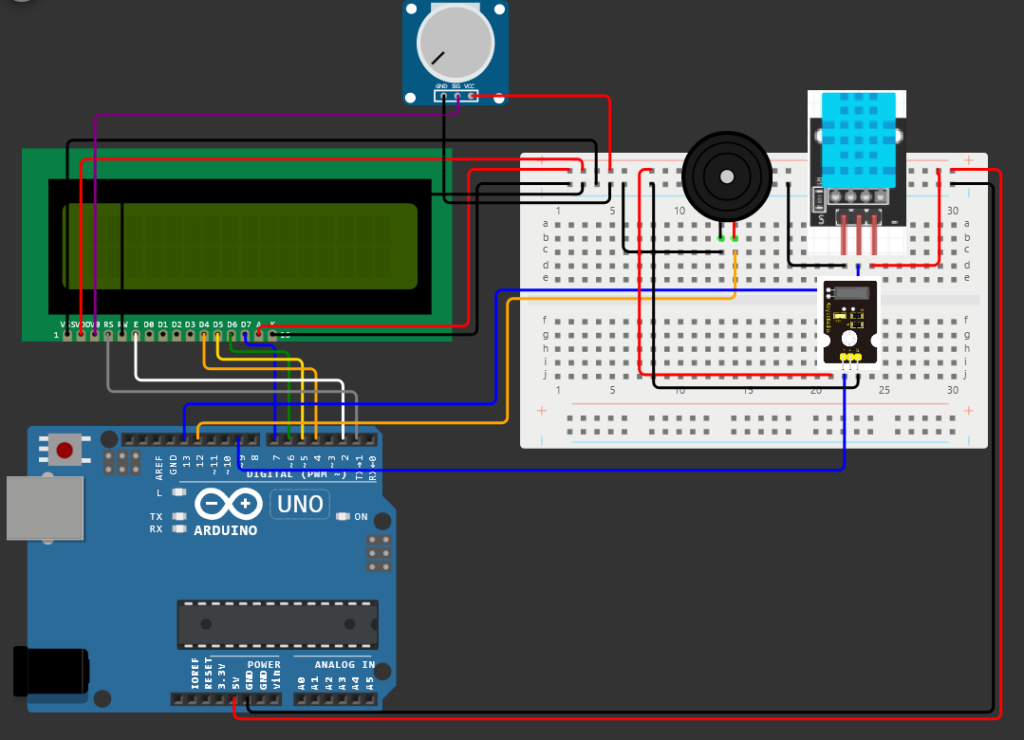
Leave a Reply