For my film prop, I decided to create a storm detection and warning system. I decided on this after looking at some of the problems plaguing Mars.
Mars suffers from heavy storms featuring fast-moving winds of speeds of up to almost 70mph and a thick, suffocating cloud of dust. It’s not something you’d want to be caught up in while unprotected on the surface, so I created a warning system.
Initial Design
My initial design for the storm detector was to use a dust sensor that can detect fine particles in the air. However, the models I found were either too expensive or wouldn’t have arrived in a reasonable time. Instead, I decided to use sensitive flex sensors to catch the wind and report wind strength based on how much they were flexed.
I ended up using a flex sensor (which the company claimed was the type used in the Nintendo Power Glove!)
My setup for the flex sensor is shown below. The sensor itself leads off a black and white wire and extends out of the shot.
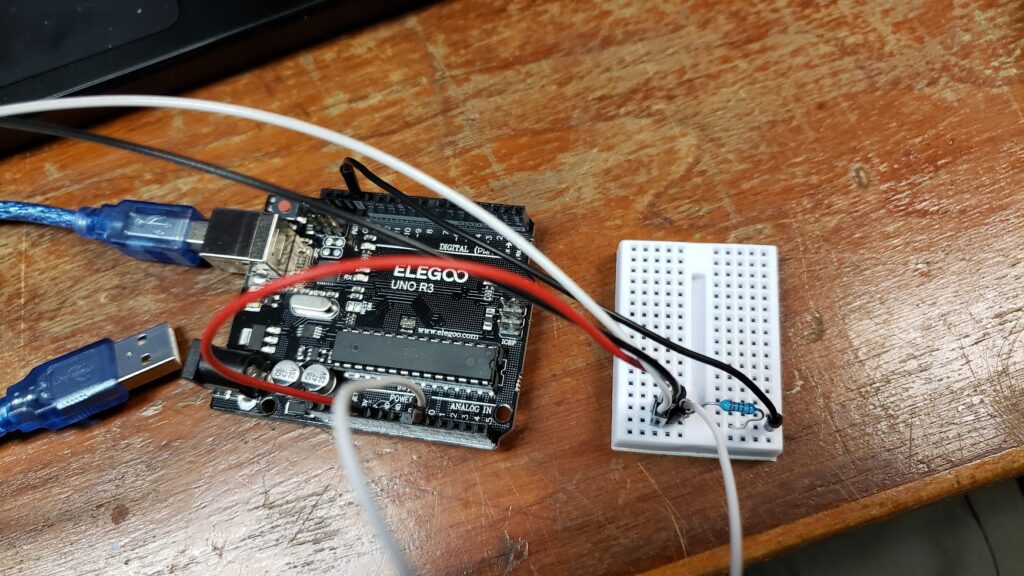
I set up my project over the prototyping breadboard as it was small enough to fit in my final container.
Flex sensors work by having a layer of conductive particles on one side. As you bend the sensor, the conductive particles move apart, causing the resistance flowing through the sensor’s body to increase. Two images from SparkFun demonstrate this principle below:
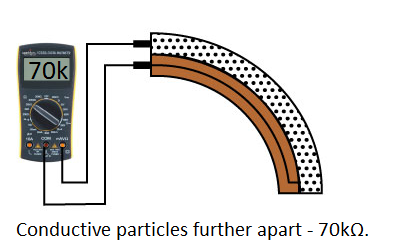
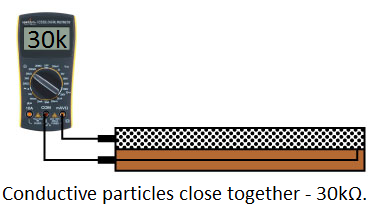
In order to have a concrete output I added a buzzer, and ended up rubber-banding the breadboard to the Arduino.
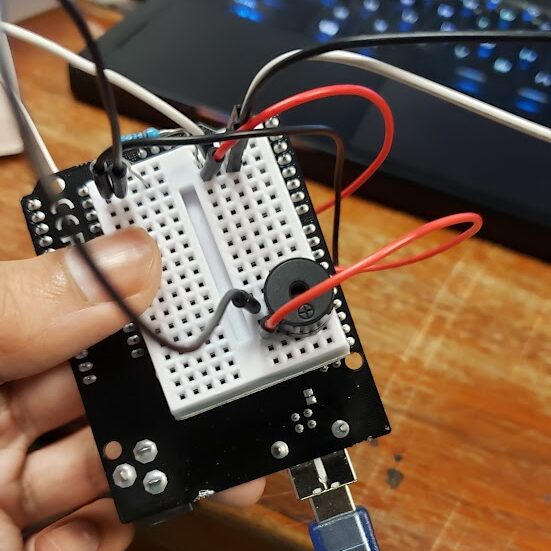
Function
The flex sensor responds with a resistance, so I wrote code that compares the resistance and bend to specific thresholds and outputs a beep once met. The higher the bend, the higher pitch the beep.
Here is a video of it at work, the data is output to serial monitor for easy viewing:
Because the sensor was so finicky, I decided to implement a rolling average in my code. This meant that the data being compared was over 10 measurements, meaning it would fluctuate less and better represent the trend in data. Below are pictures of the serial monitor with rolling average implemented
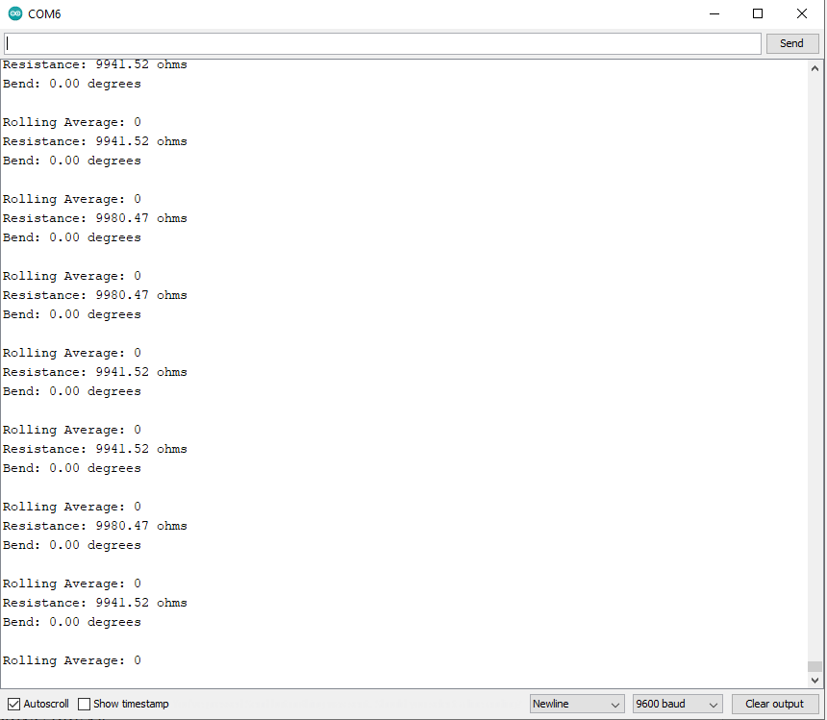
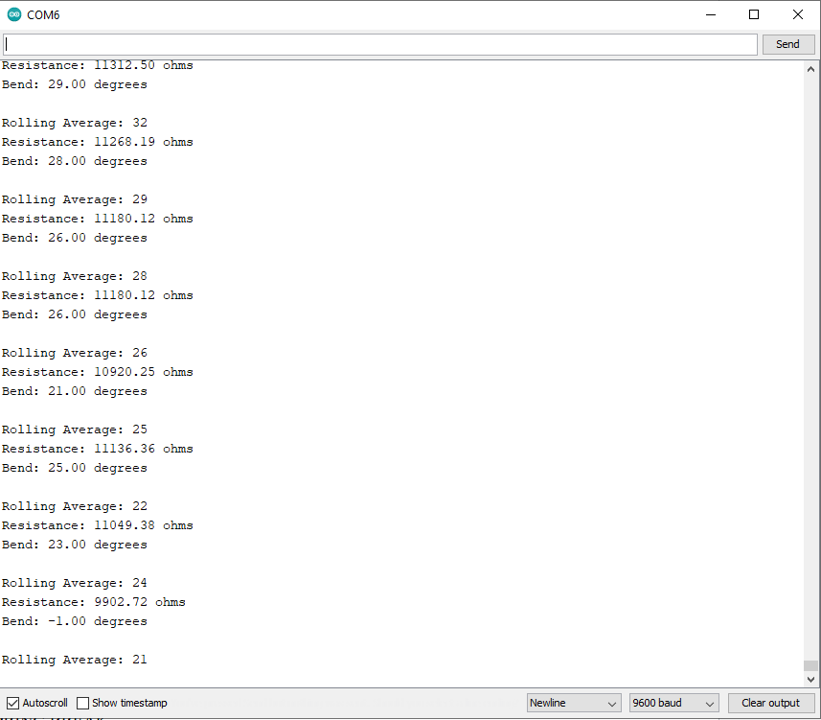
Code
/******************************************************************************
Create a voltage divider circuit combining a flex sensor with a 47k resistor.
- The resistor should connect from A0 to GND.
- The flex sensor should connect from A0 to 3.3V
As the resistance of the flex sensor increases (meaning it's being bent), the
voltage at A0 should decrease.
******************************************************************************/
const int FLEX_PIN = A0; // Pin connected to voltage divider output
// Measure the voltage at 5V and the actual resistance of your
// 47k resistor, and enter them below:
const float VCC = 4.98; // Measured voltage of Ardunio 5V line
const float R_DIV = 10000.0; // Measured resistance of 3.3k resistor
const float STRAIGHT_RESISTANCE = 9980.0; // resistance when straight
const float BEND_RESISTANCE = 14000.0; // resistance at 90 deg
int buzzerPin = 8;
const int numReadings = 10;
int readings [numReadings];
int readIndex = 0;
long total = 0;
void setup()
{
Serial.begin(9600);
pinMode(FLEX_PIN, INPUT);
pinMode(buzzerPin, OUTPUT);
tone(buzzerPin, 1000, 2000);
}
void loop()
{
// Read the ADC, and calculate voltage and resistance from it
int flexADC = analogRead(FLEX_PIN);
float flexV = flexADC * VCC / 1023.0;
float flexR = R_DIV * (VCC / flexV - 1.0);
Serial.println("Resistance: " + String(flexR) + " ohms");
// Use the calculated resistance to estimate the sensor's
// bend angle:
float angle = map(flexR, STRAIGHT_RESISTANCE, BEND_RESISTANCE,
0, 90.0);
Serial.println("Bend: " + String(angle) + " degrees");
Serial.println();
Serial.println("Rolling Average: " + String(smooth(angle)));
// series of if statements that checks thresholds of flex and plays corresponding beep
if (smooth(angle) > 2){
if (smooth(angle) > 6){
if (smooth(angle) > 10){
if (smooth(angle) > 14){
if (smooth(angle) > 22){
if (smooth(angle) > 32){
if (smooth(angle) > 44){tone(buzzerPin, 784); } // G4}
else {tone(buzzerPin, 698); } // F4}
}
else {tone(buzzerPin, 659); } // E4}
}
else {tone(buzzerPin, 587); } // D4}
}
else {tone(buzzerPin, 523); } // C4}
}
else {tone(buzzerPin, 494); } // B4
}
else {tone(buzzerPin, 440); } // A4}
}
else {noTone(buzzerPin);}
delay(500);
}
long smooth(float angle) { /* function that calculates rolling average */
////Perform average on sensor readings
long average;
// subtract the last reading:
total = total - readings[readIndex];
// read the sensor:
readings[readIndex] = angle;
// add value to total:
total = total + readings[readIndex];
// handle index
readIndex = readIndex + 1;
if (readIndex >= numReadings) {
readIndex = 0;
}
// calculate the average:
average = total / numReadings;
return average;
}
Dressed up sensor
I decided to create casing for the sensor that mimics reusable planting pots/sapling holders to fit the resourceful gardener story. A few pictures of it in the wild and a video of it in action are below.
Leave a Reply